Install phpunit
[toc]
Install PHPUnit
前置安裝:
- Composer (PHP套件管理工具)
- PHP
[(PHPUnit) [https://phpunit.de/]],PHP的測試套件,用來撰寫程式測試用。根據官網的介紹,安裝步驟如下:
- composer require --dev phpunit ^9
- ./vendor/bin/phpunit --version ## Windows 需要改寫 "./vendor/bin/phpunit" --version
1
2
3
4
5
6
7
8
9
10
11
// 調整 composer.json 後,執行 composer dump 產生 autoload.php
{
"autoload": {
"psr-4": {
"App\\":"src"
}
},
"require-dev": {
"phpunit/phpunit": "9"
}
}
PHPUnit官網上的範例程式碼
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
// test/EmailTest.php
<?php
use App\Email;
use PHPUnit\Framework\TestCase;
class EmailTest extends TestCase
{
public function testCanBeCreatedFromValidEmailAddress(): void
{
$this->assertInstanceOf(
Email::class,
Email::fromString('user@example.com')
);
}
public function testCannotBeCreatedFromInvalidEmailAddress(): void
{
$this->expectException(InvalidArgumentException::class);
Email::fromString('invalid');
}
public function testCanBeUsedAsString(): void
{
$this->assertEquals(
'user@example.com',
Email::fromString('user@example.com')
);
}
}
//src/Email.php
<?php declare (strict_types = 1);
namespace App;
class Email
{
private $email;
private function __construct(string $email)
{
$this->ensureIsValidEmail($email);
$this->email = $email;
}
public static function fromString(string $email): self
{
return new self($email);
}
public function __toString(): string
{
return $this->email;
}
private function ensureIsValidEmail(string $email): void
{
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
throw new \InvalidArgumentException(
sprintf(
'"%s" is not a valid email address',
$email
)
);
}
}
}
Running PHPUnit
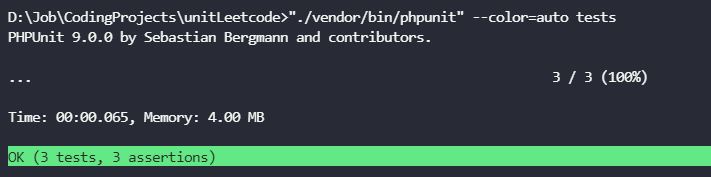
本站所有文章除特别聲明外,均採用 CC BY-SA 4.0 協議 ,轉載請註明出處!